In the DevOps world, you are continually parsing, searching, and changing the text and json files; why not automate them as Python read json files efficiently. Reading the Json and text file with Python is fun.
In this tutorial, you will learn how Python read json files and read text files with python with a few lines of code.
Still Interested? Let’s begin!
Table of Content
- Prerequisites
- Read text file and Write to text file using Pathlib python
- Read text file python and Write to text file python Using open() python function
- How Python read json file and Python write json to file
- Conclusion
Prerequisites
- Python v3.6 or later installed on your local machine. This tutorial will be using Python v3.9.2 on a Windows 10 machine.
- A code editor. Even though you can use any text editor to work with Python files. This tutorial will be using Visual Studio (VS) Code.
Read text file and Write to text file using Pathlib python
Let’s kick off this tutorial by reading and writing the text in a text file using Python. There are tons of times when you need to read the specific text from a file or the complete file, and nothing could be better than using the Pathlib python module.
Python’s standard library is very extensive, offering a wide range of facilities. The library contains built-in modules (written in C) that provide access to system functionality such as file I/O that would otherwise be inaccessible to Python programmers, as well as modules written in Python that provide standardized solutions for many problems that occur in everyday programming.
Modules are simply files containing Python functions that can be imported inside another Python Program. In simple terms, module is similar to code library or a file that contains a set of functions that you want to include in your application.
Python Library ⮕ Python Modules ⮕ Python functions
Pathlib python module offers classes representing filesystem paths with semantics appropriate for different operating systems.
Enough theory! Let’s learn how to use the Pathlib Python module practically.
- Create a Python folder in any location on your Windows machine and import the folder into the visual studio code.
- Open your favorite code editor such as visual studio code, and open the Python folder in the visual studio that you created in the previous step.
- Create a file named text.txt under the Python folder and add a text in it as “Hello, I am Tech Blogger.”
- Create another file named pathlib_module.py Copy/paste the following Python script into the file and save it.
The code snippet below uses the pathlib module to open a test.txt file from a defined path, reads the content using path.read_text()
function, and stores the content in the variable named cont. Also, path.write_text()
the function appends the text (LOG: information technology) in the same test.txt file.
The Path.write_text() the function opens a text file in text mode, writes to text file the data and closes the file. Lastly using print()
function, it prints the value of both cont and cont1.
import pathlib # Pathlib is one of the standard library of Python
# The pprint module is used to “pretty-print” Python code which can be further used as input to the interpreter
from pprint import pprint
path = pathlib.Path("test.txt")
cont = path.read_text()
cont1 = path.write_text("LOG:information technology")
print(cont)
# cont1 provides the number of indexes used in the text "LOG:information technology"
print(cont1)
- Now, execute the program using the Python command you should see the output below. After the python code is executed, you will notice that it will read the content from file text.txt and then print it. Also, the write method overrides the content into the file and then prints the number of index of the content in the file, which is 26 [LOG: information technology]
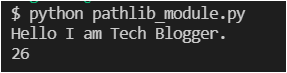
- Also, you should see the content in the file has been overridden from Hello I am Tech Blogger to LOG: information technology.
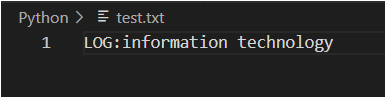
Read text file python and Write to text file python Using open() python function.
In the previous section, you learned the first way to read and write text in text files using the pathlib python module, but there are many ways to do that, and one of the other great ways is by using the open() function. Let’s jump into it and learn.
With the open() python function, you open the text file to perform operations such as read, append data, close the file, etc.
- Create another file named open_function.py and Copy/paste the following Python script into the file and save it.
As soon as the file is opened, the below code reads the file using read()
function and stores the values in the variable named content. Further, it prints the content and the length of the file’s content using print() the function and closes the file.
myfile = open('test.txt')
content = myfile.read()
print(content)
print(len(content))
myfile.close() # Always close your file so that it doesnt consume memory

Let’s check out another example where mode is defined as r
and w
which allows you to read the content and write to append the data in the file.
The Difference between
w
anda
mode is w overwrites the data in file and a appends the data in the file. Also, If you intend to open any binary file such as pdf use the mode asrb
orwb
which denotes read or write binary.
- Create another file named open_function2.py and Copy/paste the following Python script into the file and save it.
The code below opens a file, reads the content using read() the function, and prints the content. Further, write the content in the file “Adding new line” using write() the function.
with open("test.txt",mode="r") as mynewfile:
contents2 = mynewfile.read()
print(contents2)
with open("test.txt",mode="w") as mynewfile:
contents1 = mynewfile.write("Adding new Line")

How Python read json file and Python write json to file
You have learned how to work with text files with various methods in Python, but at times, you need to work with JSON files as well, and python continues to be great for working with JSON files. In this section, let’s learn how Python read json file and Python write json to files with two functions json.load()
and json.dump().
JSON module helps to work with JSON files such as reading a JSON file, writing data in JSON file or appending the file, etc. json.load() the function is used to open a JSON file. json.dump() the function will add data into the JSON file.
- Create another file named file.json, and Copy/paste the following text into the file and save it.
{
"automate": 1,
"infra": 2
}
- Create another file named json_code.py, copy/paste the following Python script into the file and save it.
- The below code will perform the below steps in different parts as described below:
- Opens and reads a JSON file using json.load() described in part 1
- Updates the file but only in outputs, not in actual memory described in part 2
- Finally updates the file in outputs and actual memory using json.dump() described in part 3.
import json
from pprint import pprint
# Part 1: Opening and Reading a JSON file using json.load()
with open('file.json', 'r') as opened_file: # Opens a Json File
policy = json.load(opened_file)
pprint(policy)
# Part 2: Updating the file but only in outputs not in actual memory
policy['c'] = '3'
pprint(policy)
# Part 3: Updating the file in outputs as well in actual memory using json.dump()
with open('file.json', 'w') as opened_file1:
policy2 = json.dump(policy, opened_file1)
pprint(policy2)
with open('file.json', 'r') as opened_file2: # Opens a Json File
policy3 = json.load(opened_file2)
pprint(policy3)

Conclusion
In this tutorial, you learned how to read write text within text files and JSON files. Automating with Python simplifies the tasks with few lines of code and keeps you away from silly manual errors.
With that now, you have sound knowledge of reading and writing to text files in python, so what do you plan next to read json files or text files with python?